Email: b4borad74@gmail.com Contact: +91 9724412437
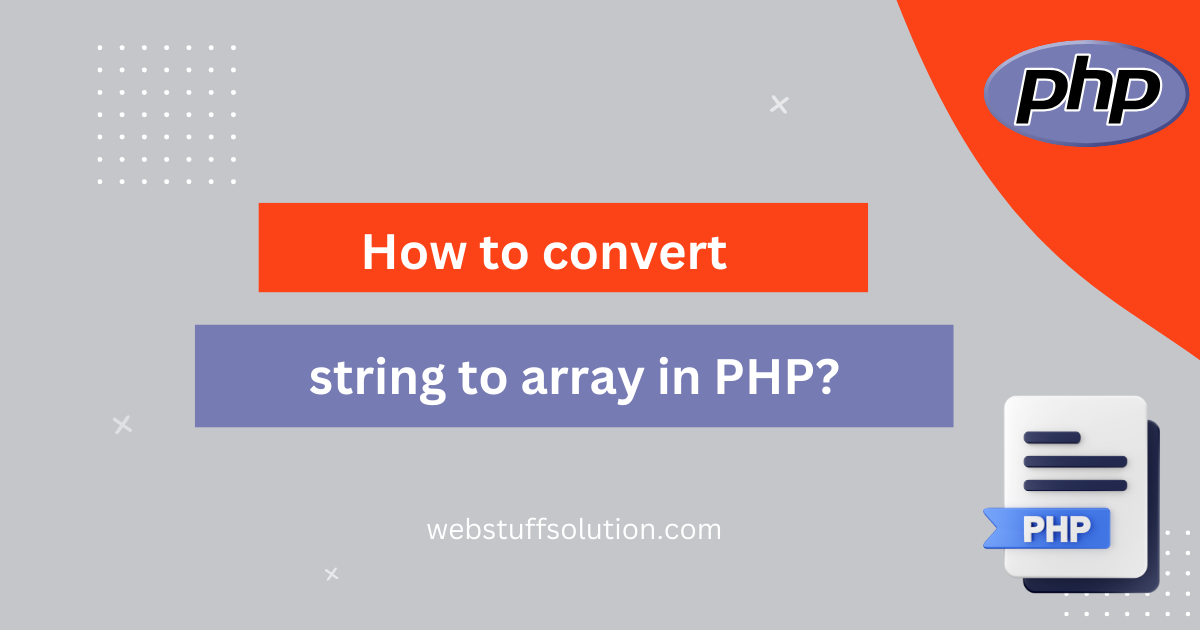
How to convert string to array in PHP?
ByJasminkumar Borad
- On
- InPHP
In this article, you’ll learn how to convert string to array in PHP, with a focus on using a comma as the delimiter. We’ll explore various methods for performing this conversion.
Arrays are often more suitable than strings in many scenarios. For instance, converting a string to an array can be useful in various situations.
Here are the methods you’ll learn about for converting a string to an array:
- ‘explode()’ function
- ‘preg_split()’ function
- ‘json_decode()’ function
- ‘str_split()’ function
How to convert string to array in PHP?
1. explode() function
We will use the ‘explode()’ function to convert a string to an array in PHP. The ‘explode()’ function provides a straighrforward method to perform this conversion. I’ll provide multiple examples to help you understand how it works.
Let’see the example below.
<?php
$myNewString = "Hi How are you";
$new_array = explode(' ', $myNewString);
print_r($new_array);
?>
Output:
Array
(
[0] => Hi,
[1] => How
[2] => are
[3] => you
)
Read also: PHP Remove last character from string
2. preg_split() function
preg_split() is a function that split a string using a regular expression.
Syntax
preg_split($pattern, $subject, $limit, $flags)
<?php
$myNewString = preg_split("/[\s,]/", "Hi How are you ");
print_r($myNewString);
?>
Output:
Array
(
[0] => Hi
[1] => How
[2] => are
[3] => you
)
3. json_decode() function
The json_decode() function is used to decode a JSON encoded string. JSON string usually represents the objects into data-value pairs.
<?php
$json_value = '{"id" : 1, "total_id" : 100, "max_id" : 200}';
$new_array = json_decode($json_value, true);
print_r($new_array);
?>
Output:
Array
(
[id] => 1
[total_id] => 100
[max_id] => 200
)
4. str_split() function
This is an in-built PHP method that is used to convert string to array in PHP. It does not use any kind of separator, just splits the string.
<?php
$myNewString = "Hi How are you";
$new_array = str_split($myNewString, 3);
print_r($new_array);
?>
Output:
Array
(
[0] => Hi
[1] => How
[2] => ar
[3] => e y
[4] => ou
)
I hope this tutorial help you.